Step by Step Guide to Integrate Gooogle Maps API in Angular Project
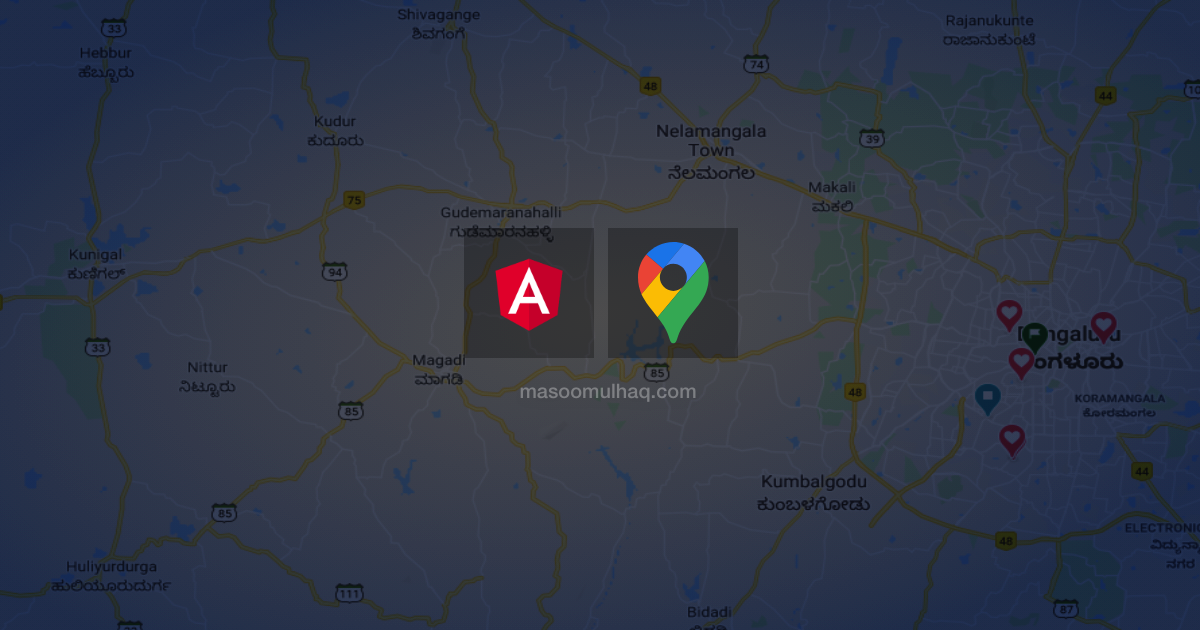
In this article, we will be learning how to integrate Google Maps API in your Angular project.
Before we start, I assume you have all these ready:
- Angular CLI
- An existing Angular project
Step 1: Install AGM
|
|
This will install the Angular Google Maps (or AGM) dependency. We require an API key before we integrate AGM with our Angular App. So let’s create it first.
Step 2: Create Google Maps API Key
To create an API Key, you must log in into the Google Cloud Platform Console and create a project first. If the project already exists, select the respective project from the Select a Project dropdown.
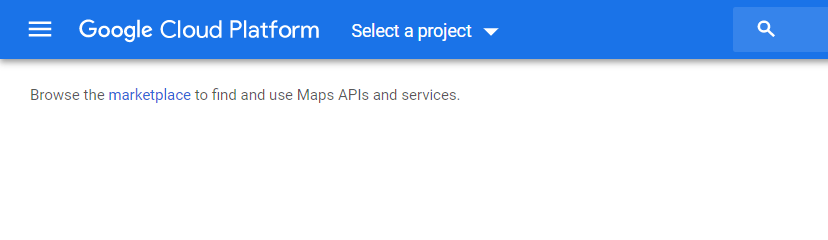
Select a Project
In case, if you want to create a new one. Click on New Project button as shown in the below example.
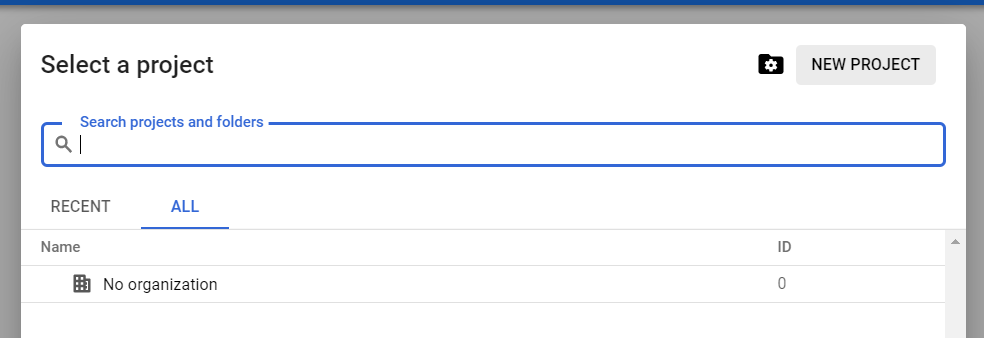
Create a New Project (If not created)
Once the project is created, click on your project -> then click on Open button at the bottom right in the dialog.
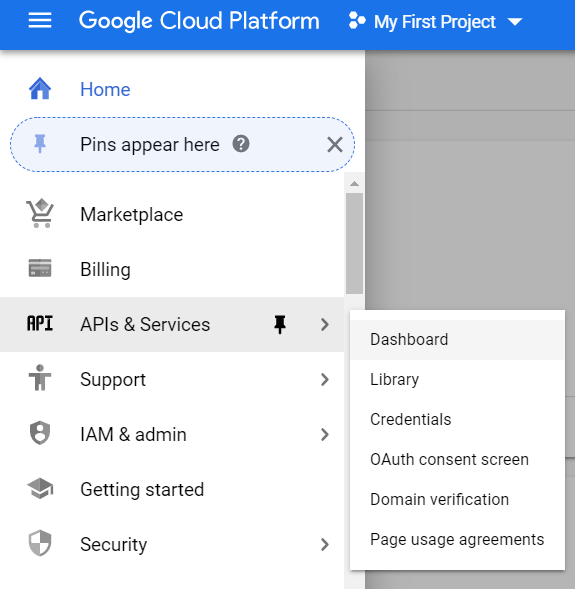
Click on APIs & Services -> Credentials
You will be asked to create credentials. Click on Create a credentials button and select the API key from the dropdown. This will open up a dialog with a new API key. Copy-Paste it in your notepad for the time being.
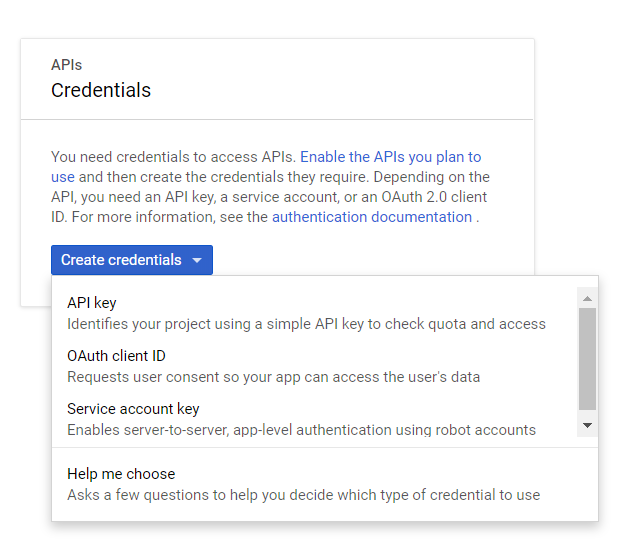
Click on Create a credentials -> API key
This is the final step, search for “Maps JavaScript API” in the main search bar, you will be taken the library page where you will be asked to enable the library. Click on Enable button to do the same.
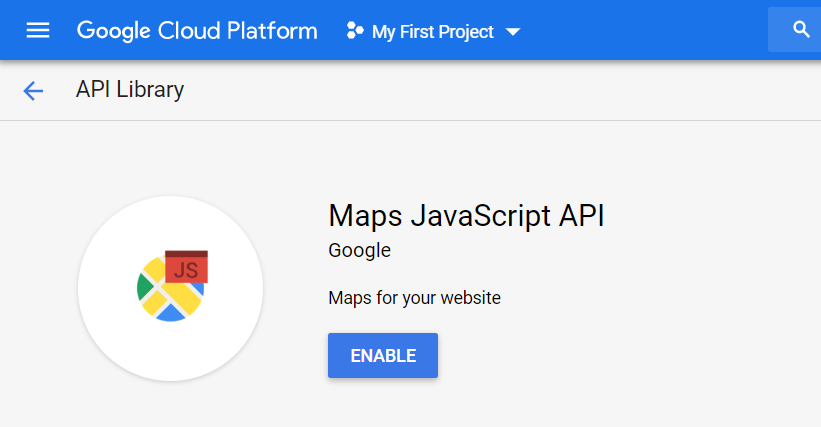
Enable Maps JavaScript API
First 1 million requests are free and then you will be charged based on the usage. To make it production-ready you need to enable billing. Here is the reference.
Now you are ready to integrate AGM.
Step 3: Include AGM in your Angular Project
|
|
Include AgmCoreModule
in your app.module.ts
and paste your newly generated key as in the above example. For more information or customization refer here.
All set! Now we are ready to test it out.
Final Step: Run an example
Here is how *.component.ts
file should look like, modify accordingly:
|
|
and the template *.component.html
should be like:
|
|
and here is the mandatory thing required, we must give mandatory height to agm-map
to make the maps visible:
|
|
That’s it! Hope you like this article. In case of any queries, comment out below. Feel free to share with others.